Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 보안
- php artisan
- 카카오가고싶다
- 기타정리
- Backend
- 복습 #회사
- 작업물
- 코딩테스트
- 개인공부
- 작업물 #영상편집 #서브컬쳐
- centOS
- centOS7
- AWS
- NGINX
- ratchet
- 일상
- linux #centos
- 에러해결
- php-fpm
- laravel
- vagrant
- jquery
- php
- MySQL
- 키워드
- 키워드 정리
- error
- 시벌이슈
- 네이버싫어
- 메모
Archives
- Today
- Total
개발을 간바루Joy 하게
[PHP] Ratchet Websocket 설치 및 사용방법 본문
※해당글은 composer 설치가 되있음을 가정합니다.
서버1 환경
OS : Centos7
php : php7.4
서버 2 환경
OS : Amazon Linux 2
php : php7.2
Ratchet 라이브러리는 php 5.4 이상 버전만 사용 가능합니다.
우선 composer로 ratchet 라이브러리를 다운받습니다
composer require cboden/ratchet
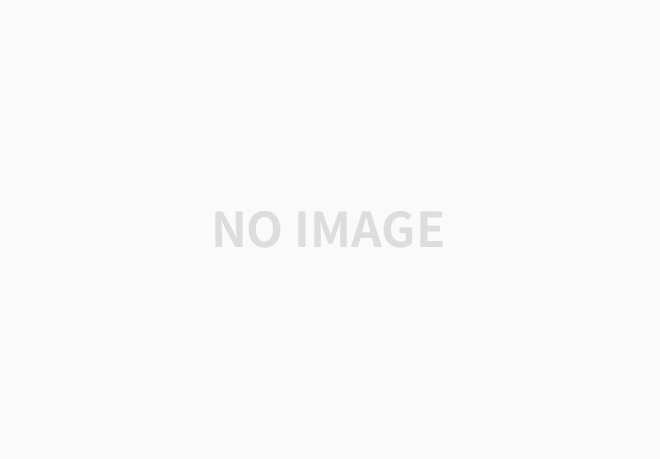
정상적으로 설치가 되었다면 composer.json파일에 다음구문이 생성됩니다.
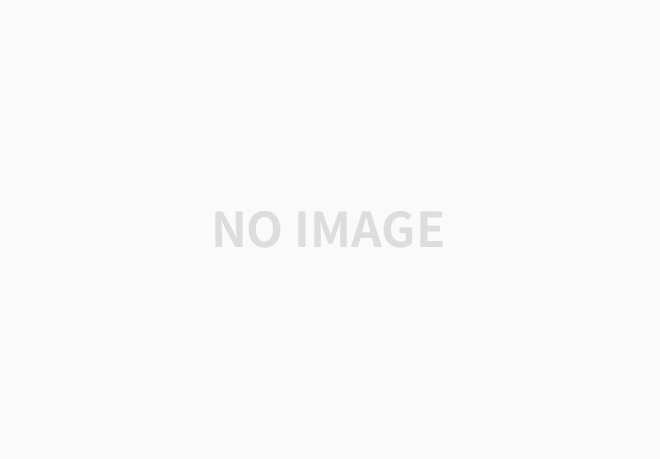
Class 파일들을 NameSpace묶어서 사용하기 위해 json파일을 열어 동그라미친 부분을
"네임스페이스 이름\\": "파일경로/"
이런식으로 변경을 해줍니다.
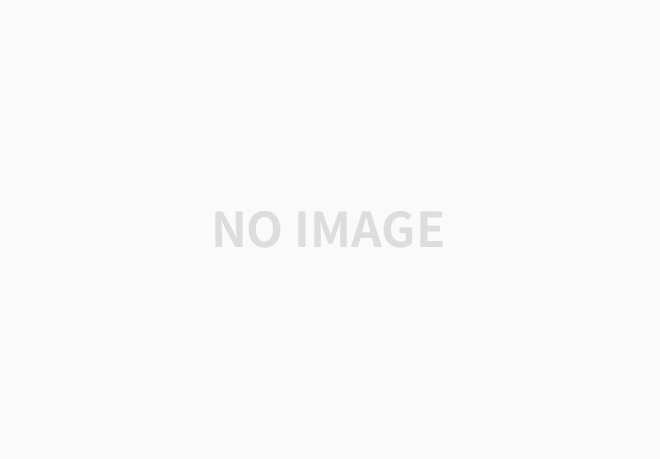
composer update
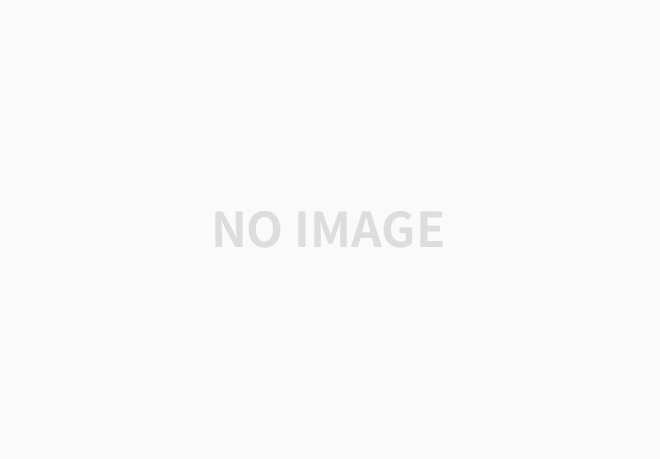
웹소켓 로직
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
<?php
//composer.json에서 설정한 namespace
namespace ServerClass;
use Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
class RatchetSocket implements MessageComponentInterface {
protected $clients;
public function __construct() {
// clients 변수에 접속 클라이언트들을 담을 객체 생성
$this->clients = new \SplObjectStorage;
}
// 클라이언트 접속
public function onOpen(ConnectionInterface $conn) {
// clients 객체에 클라이언트 추가
$this->clients->attach($conn);
echo "New connection! ({$conn->resourceId}) / Clients Count : {$this->clients->count()}\n";
}
//메세지 전송, $from 인자값은 메세지를 보낸 클라이언트의 정보, $msg인자값은 보낸 메세지
public function onMessage(ConnectionInterface $from, $msg) {
//접속한 clients 수만큼 반복
foreach ($this->clients as $client) {
//접속한 클라이언트 본인 이외일때
if ($from !== $client) {
//메세지 전송
$client->send($msg);
}
}
}
//클라이언트 접속 종료
public function onClose(ConnectionInterface $conn) {
// clients 객체에 클라이언트 접속정보 제거
$this->clients->detach($conn);
echo "Connection {$conn->resourceId} has disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "An error has occurred: {$e->getMessage()}\n";
$conn->close();
}
}
|
cs |
웹소켓 실행 로직
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
<?php
use Ratchet\Server\IoServer;
use Ratchet\Http\HttpServer;
use Ratchet\WebSocket\WsServer;
// namespace\class 이름
use ServerClass\RatchetSocket;
//ratchet 오토로더
require __DIR__. '/vendor/autoload.php';
$server = IoServer::factory(
new HttpServer(
new WsServer(
new RatchetSocket()
)
),
8090
);
$server->run();
|
cs |
클라이언트 로직
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8"/>
</head>
<body>
<div style="margin:0 auto; width: 500px;">
<input type="text" id="message" value="" />
<button onclick="sendData();">Send</button>
</div>
</body>
<script>
// Create a new WebSocket.
var socket = new WebSocket('ws://{아이피주소}:{포트번호}/pos/webSocketRun.php');
var message = document.getElementById('message');
// Define the
socket.onopen = function(e) {
console.log("Connection established!");
};
//받는 데이터
socket.onmessage = function(e) {
console.log(e.data);
}
//보내는 데이터
function sendData(){
var sendData = document.querySelector("#message").value;
socket.send(sendData);
}
</script>
</html>
|
cs |
웹소켓 실행
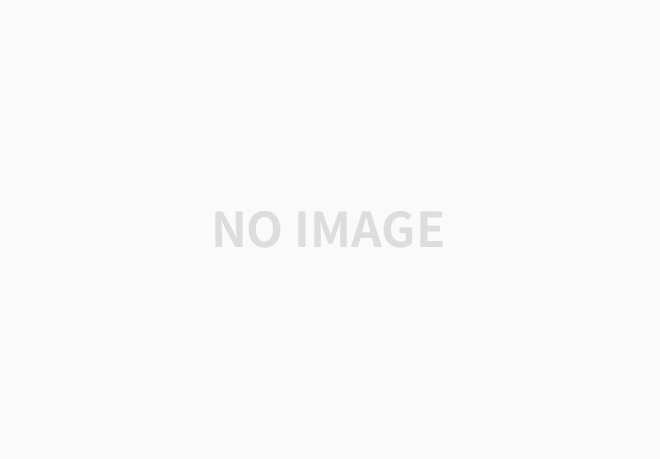
클라이언트 접속
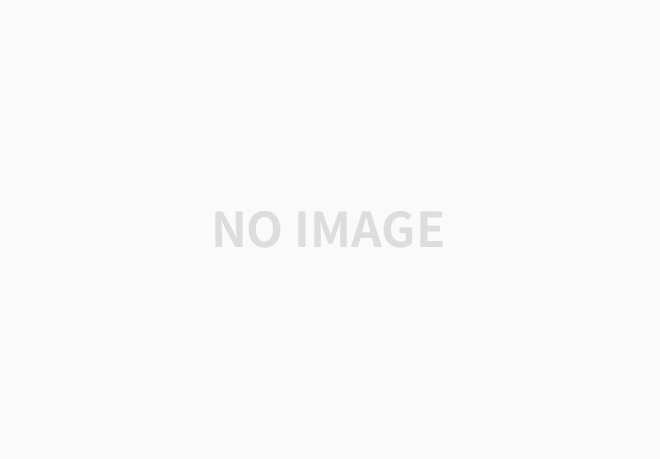
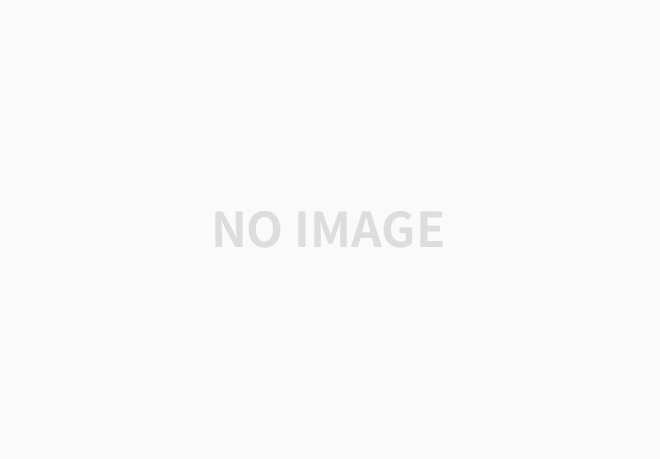
메세지 전송 테스트
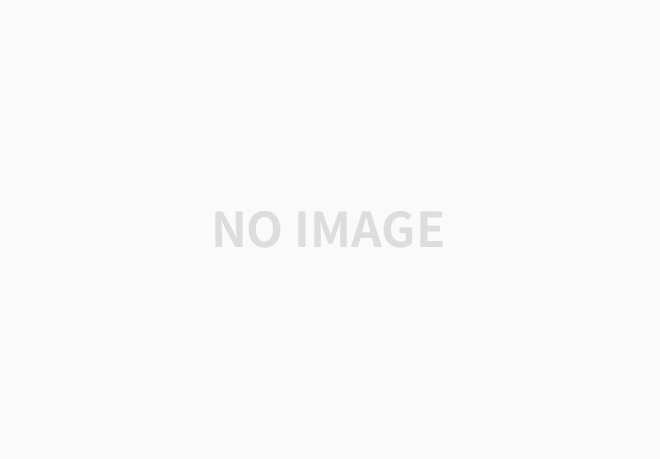
※추가 웹소켓 다수 포트open 로직
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
<?php
use Ratchet\Server\IoServer;
use Ratchet\Http\HttpServer;
use Ratchet\WebSocket\WsServer;
// namespace\class 이름
use ServerClass\RatchetSocket;
//다수포트 사용시 추가
use React\Socket\Server;
use React\EventLoop\Factory as EventLoopFactory;
$chatServer = new RatchetSocket;
$loop = EventLoopFactory::create();
$ports = [8090, 8091, 8092, 8093, 8094, 8095, 8096, 8097,8098, 8099];
foreach($ports as $port){
$sock = new Server("아이피주소:{$port}", $loop);
$server = new IoServer(
new HttpServer(
new WsServer($chatServer)
),
$sock,
$loop
);
}
$loop->run();
|
cs |
'프로그래밍 > PHP' 카테고리의 다른 글
[PHP] Websocket Ratchet Client 사용하기 (0) | 2021.10.28 |
---|---|
[PHP] Socket Server Code (0) | 2021.10.19 |
[PHP] Ratchet WebSocket Connection limit 이슈 (0) | 2021.09.09 |
[PHP] 엑셀 csv 파일 가공 (0) | 2021.05.04 |
DeZend (PHP디코딩) 사용방법 (0) | 2021.04.14 |